A library of over 250,000 device drivers, firmware, BIOS and utilities for Windows. On Windows 10, a device driver is an essential piece of code, which allows the system to interact with a specific hardware (such as graphics card, storage driver, network adapter, Bluetooth, etc.


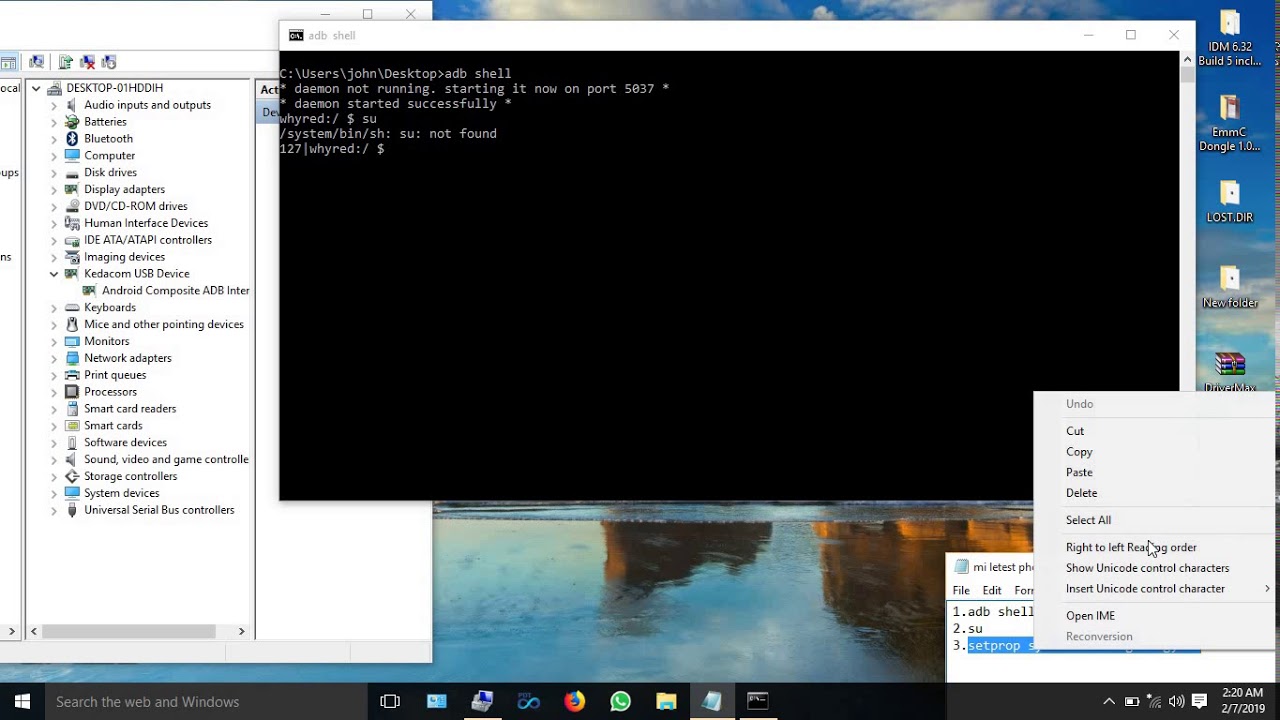
A USB device exposes its capabilities in the form of a series of interfaces called a USB configuration. Each interface consists of one or more alternate settings, and each alternate setting is made up of a set of endpoints. This topic describes the various descriptors associated with a USB configuration.
A USB configuration is described in a configuration descriptor (see USB_CONFIGURATION_DESCRIPTOR structure). A configuration descriptor contains information about the configuration and its interfaces, alternate settings, and their endpoints. Each interface descriptor or alternate setting is described in a USB_INTERFACE_DESCRIPTOR structure. In a configuration, each interface descriptor is followed in memory by all of the endpoint descriptors for the interface and alternate setting. Each endpoint descriptor is stored in a USB_ENDPOINT_DESCRIPTOR structure.
For example, consider a USB webcam device described in USB Device Layout. The device supports a configuration with two interfaces, and the first interface (index 0) supports two alternate settings.
The following example shows the configuration descriptor for the USB webcam device:
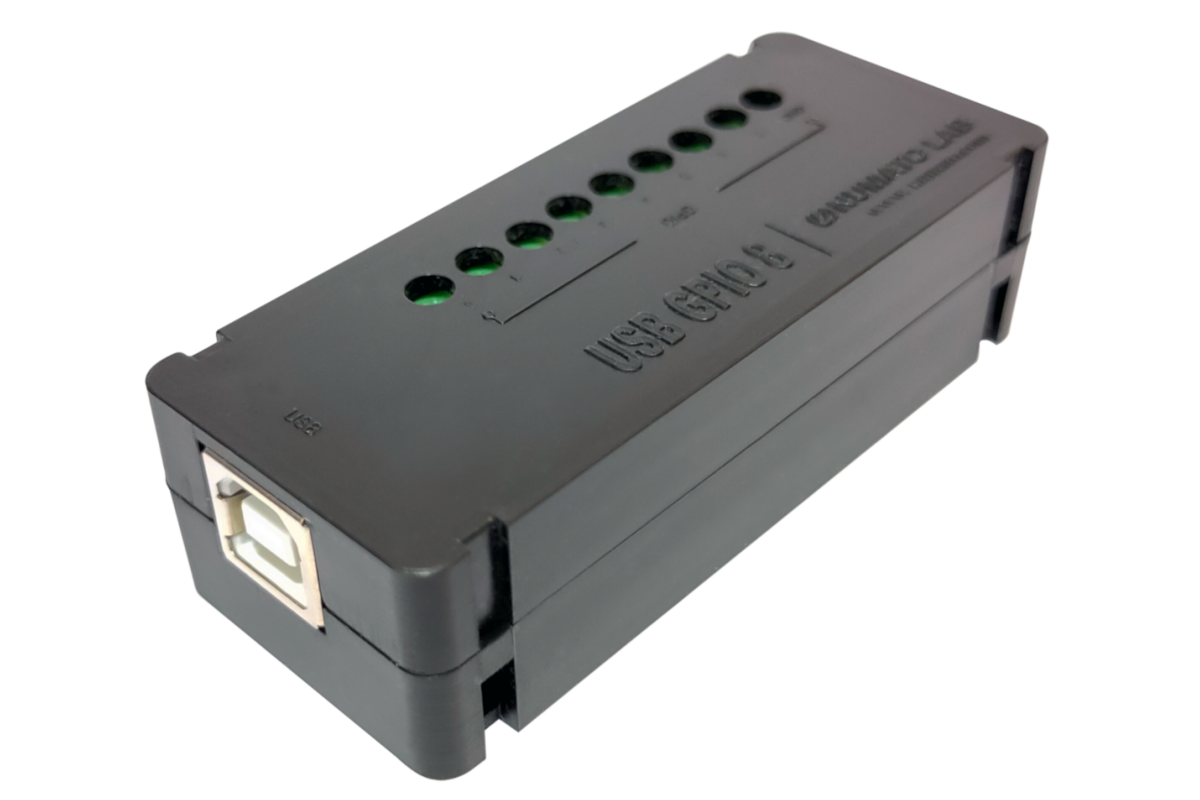
The bConfigurationValue field indicates the number for the configuration defined in the firmware of the device. The client driver uses that number value to select an active configuration. For more information about USB device configuration, see How to Select a Configuration for a USB Device. A USB configuration also indicates certain power characteristics. The bmAttributes contains a bitmask that indicates whether the configuration supports the remote wake-up feature, and whether the device is bus-powered or self-powered. The MaxPower field specifies the maximum power (in milliamp units) that the device can draw from the host, when the device is bus-powered. The configuration descriptor also indicates the total number of interfaces (bNumInterfaces) that the device supports.
The following example shows the interface descriptor for Alternate Setting 0 of Interface 0 for the webcam device:
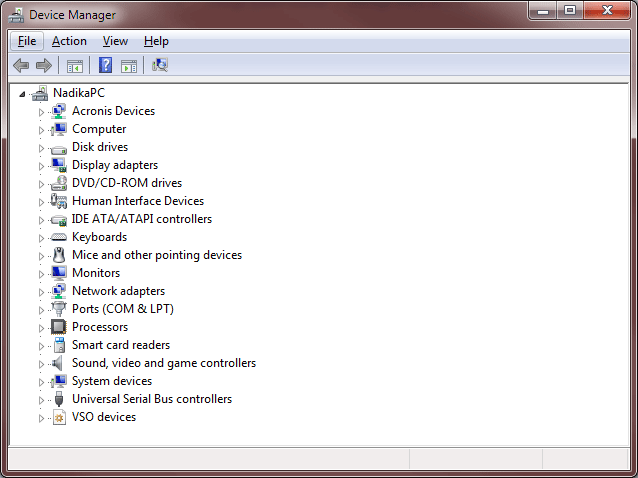
In the preceding example, note bInterfaceNumber and bAlternateSetting field values. Those fields contain index values that a client driver uses to activate the interface and one of its alternate settings. For activation, the driver sends a select-interface request to the USB driver stack. The driver stack then builds a standard control request (SET INTERFACE) and sends it to the device. Note the bInterfaceClass field. The interface descriptor or the descriptor for any of its alternate settings specifies a class code, subclass, and protocol. The value of 0x0E indicates that the interface is for the video device class. Also, notice the iInterface field. That value indicates that there are two string descriptors appended to the interface descriptor. String descriptors contain Unicode descriptions that are used during device enumeration to identify the functionality. For more information about string descriptors, see USB String Descriptors.
Each endpoint, in an interface, describes a single stream of input or output for the device. A device that supports streams for different kinds of functions has multiple interfaces. A device that supports several streams that pertain to a function can support multiple endpoints on a single interface.
All types of endpoints (except the default endpoint) must provide endpoint descriptors so that the host can get information about endpoint. An endpoint descriptor includes information, such as its address, type, direction, and the amount of data the endpoint can handle. The data transfers to the endpoint are based on that information.
The following example shows an endpoint descriptor for the webcam device:
The bEndpointAddress field specifies the unique endpoint address that contains the endpoint number (Bits 3.0) and the direction of the endpoint (Bit 7). By reading those values in the preceding example, we can determine that the descriptor describes an IN endpoint whose endpoint number is 2. The bmAttributes attribute indicates that the endpoint type is isochronous. The wMaxPacketSizefield indicates the maximum number of bytes that the endpoint can send or receive in a single transaction. Bits 12.11 indicate the total number of transactions that can be sent per microframe. The bInterval indicates how often the endpoint can send or receive data.
How to get the configuration descriptor
The configuration descriptor is obtained from the device through a standard device request (GET_DESCRIPTOR), which is sent as a control transfer by the USB driver stack. A USB client driver can initiate the request in one of the following ways:
If the device supports only one configuration, the easiest way is to call the framework-provided WdfUsbTargetDeviceRetrieveConfigDescriptor method.
For a device that supports multiple configurations, if the client driver wants to get the descriptor of the configuration other than the first, the driver must submit an URB. To submit an URB, the driver must allocate, format, and then submit the URB to the USB driver stack.
To allocate the URB, the client driver must call the WdfUsbTargetDeviceCreateUrb Viewsonic driver download for windows 10 pro. method. The method receives a pointer to an URB allocated by the USB driver stack.
To format the URB, the client driver can use the UsbBuildGetDescriptorRequest macro. The macro sets all the necessary information in the URB, such as the device-defined configuration number for which to retrieve the descriptor. The URB function is set to URB_FUNCTION_GET_DESCRIPTOR_FROM_DEVICE (see _URB_CONTROL_DESCRIPTOR_REQUEST) and the type of descriptor is set to USB_CONFIGURATION_DESCRIPTOR_TYPE. By using the information contained in the URB, the USB driver stack builds a standard control request and sends it to the device.
To submit the URB, the client driver must use a WDF request object. To send the request object to the USB driver stack asynchronously, the driver must call the WdfRequestSendmethod. To send it synchronously, call the WdfUsbTargetDeviceSendUrbSynchronously method.
WDM drivers: **A Windows Driver Model (WDM) client driver can only get the configuration descriptor by submitting an URB. To allocate the URB, the driver must call the USBD_UrbAllocate routine. To format the URB, the driver must call the UsbBuildGetDescriptorRequest** macro. To submit the URB, the driver must associate the URB with an IRP, and submit the IRP to the USB driver stack. For more information, see How to Submit an URB.
Within a USB configuration, the number of interfaces and their alternate settings are variable. Therefore, it's difficult to predict the size of buffer required to hold the configuration descriptor. The client driver must collect all that information in two steps. First, determine what size buffer required to hold all of the configuration descriptor, and then issue a request to retrieve the entire descriptor. A client driver can get the size in one of the following ways:
To obtain the configuration descriptor by calling WdfUsbTargetDeviceRetrieveConfigDescriptor, perform these steps:
- Get the size of buffer required to hold all of the configuration information by calling WdfUsbTargetDeviceRetrieveConfigDescriptor. The driver must pass NULL in the buffer, and a variable to hold the size of the buffer.
- Allocate a larger buffer based on the size received through the previous WdfUsbTargetDeviceRetrieveConfigDescriptor call.
- Call WdfUsbTargetDeviceRetrieveConfigDescriptor again and specify a pointer to the new buffer allocated in step 2.
To obtain the configuration descriptor by submitting an URB, perform these steps:
- Allocate an URB by calling the WdfUsbTargetDeviceCreateUrb method.
- Format the URB by calling the UsbBuildGetDescriptorRequest macro. The transfer buffer of the URB must point to a buffer large enough to hold a USB_CONFIGURATION_DESCRIPTOR structure.
- Submit the URB as a WDF request object by calling WdfRequestSend or WdfUsbTargetDeviceSendUrbSynchronously.
- After the request completes, check the wTotalLength member of USB_CONFIGURATION_DESCRIPTOR. That value indicates the size of the buffer required to contain a full configuration descriptor.
- Allocate a larger buffer based on the size retrieved in wTotalLength.
- Issue the same request with the larger buffer.
The following example code shows the UsbBuildGetDescriptorRequest call for a request to get configuration information for the i-th configuration:
When the device returns the configuration descriptor, the request buffer is filled with interface descriptors for all alternate settings, and endpoint descriptors for all endpoints within a particular alternate setting. Instant videoxpress driver download for windows xp. For the device described in USB Device Layout, the following diagram illustrates how configuration information is laid out in memory.
The zero-based bInterfaceNumber member of USB_INTERFACE_DESCRIPTOR distinguishes interfaces within a configuration. For a given interface, the zero-based bAlternateSetting member distinguishes between alternate settings of the interface. The device returns interface descriptors in order of bInterfaceNumber values and then in order of bAlternateSetting values.
To search for a given interface descriptor within the configuration, the client driver can call USBD_ParseConfigurationDescriptorEx. In the call, the client driver provides a starting position within the configuration. Optionally the driver can specify an interface number, an alternate setting, a class, a subclass, or a protocol. The routine returns a pointer to the next matching interface descriptor.
Drivers Usb Config Port Devices Download
To examine a configuration descriptor for an endpoint or string descriptor, use the USBD_ParseDescriptors routine. The caller provides a starting position within the configuration and a descriptor type, such as USB_STRING_DESCRIPTOR_TYPE or USB_ENDPOINT_DESCRIPTOR_TYPE. The routine returns a pointer to the next matching descriptor.
Related topics
How to Select a Configuration for a USB Device
USB Descriptors
Qualcomm Diagnostic Mode or DIAG Mode is useful for changing the IMEI or repair baseband on your phone using QPST, WriteDualIMEI, and other tools. If you are facing 'cannot find DIAG COM Port' or similar error, then follow the steps listed in this tutorial to enable Qualcomm Diagnostic Mode or activate DIAG Mode.
Table of Contents
What is DIAG Mode?
Qualcomm DIAG Mode is a special diagnostic mode available on Qualcomm Snapdragon processor powered smartphones. When the Qualcomm Diagnostic Mode is enabled on your phone, it opens the DIAG port that helps to write or repair IMEI number using tools like QPST Tool, QFIL Tool, and other tools.
It is worth mentioning that for MediaTek (MTK) chipset powered devices, we use the Miracle Box tool to repair IMEI number, and DIAG COM Port is not required to repair IMEI on MediaTek and other processors like Spreadtrum, etc. But if you want to repair IMEI on any Qualcomm devices like Samsung, Oppo, Vivo, Xiaomi, OnePlus, Nokia, Lenovo, LG, and Motorola, then the diag port is required.
PLEASE NOTE
DIAG Port (Qualcomm HS-USB Android Diag 901D) and Qualcomm HS-USB QDLoader 9008 are separate things. Android DIAG 901D is a diag port, and HS-USB QDLoader is an EDL Mode (Emergency Download Mode).
To fix IMEI or repair baseband, it is required that your device has proper root permissions. So, make sure your device is rooted and have proper Root permissions.
How to Enable/Activate Qualcomm Diagnostic Mode on Android
There are three different methods to enable Qualcomm Diag port on your Qualcomm device. Check out all the methods below, and if one method doesn't work, then follow the other method.
[A.] Activate Qualcomm Diagnostic Mode using Diag Port Code (Dial Number)
[B.] Enable DIAG port using ADB diag Command Method
[C.] Activate Diag port using Terminal Emulator App (APK)
Enable Diag Mode using Secret Code
Some phones support activating Diag mode using the secret code. Follow the below steps to see if this method works on your device.
Open phone dialer app on your device and type #*#717717#*#*
. It should enable Diag Mode, and fix cannot find DIAG COM Port error. If nothing happens, then try other methods listed below.
Enable / Activate Diag Mode from ADB
In this method, we will make use of ADB commands to enable Diag Mode. It requires that you have rooted your device and have proper root permissions.
- Install ADB and Fastboot drivers on your PC from here. Also, make sure to install the suitable Android USB Driver for your device model.
- Enable USB Debugging on your device from Settings > Developer Options. If Developer Options are missing, enable them from Settings > About Phone and tap on Build Number for about 7-10 times.
- Connect your device to PC and enter the below command.
This command lists the devices connected to PC with USB Debugging enabled. If your device doesn't show up in the list, then make sure you have installed ADB, Fastboot, and USB Drivers properly.
- Now, type the below command and hit enter:
This command enables Android Debug Shell. Using ADB Shell, we can perform several system actions.
- Now, enter the below command in CMD window and hit enter:
This command requests superuser / Root permissions. Once the permissions are allowed, all the commands will run as the superuser.
Download the Star printer driver here.Click the link to download and then extract the folder to the desktop Double-click the Autorun.exe file inside the folder and the following window should appear: Click Installation, accept the installation agreement. STARUK.EXE(412KB) Latest Windows 3.x printer drivers for United Kingdom Market Click here for a list of the supported printers. Self-extracting archive. 1996/07/31: STARUS.EXE(773KB) Latest Windows 3.x printer drivers for American Market Click here for a list of the supported printers. Self-extracting archive. 1996/07/31: WIN95.EXE(218KB). Star Micronics Support Database. Welcome to the Star Micronics Global Support Site! Browse Star's online database to easily and quickly find drivers, software, documentation and FAQs. To begin, choose your printer below. Star Micronics Software License Agreement. 2020/12/09 - STARPRNT SDK for Windows Desktop V5.14.0 is available. 2020/12/09 - STARPRNT SDK for Windows Modern UI V5.14.0 is available. 2020/08/18 - mC-Print Utility V1.5.1 for Android has been released on Google Play. 2019/01/25 - Star mPOP online manual has been updated. 2019/01/24 - Star TSP100IIIW online manual has been updated. Drivers - Printers - Star Micronics - Download. Sep 22, 2020 Ensure the printer is not connected to the computer at this point in time. Insert the CD into the CDROM driver or click “AutoRun.exe” in the main directory to execute the installer. If the CD was misplaced, the driver can be downloaded for free from the Star Micronics Global Support Site.
- Finally, enter the below command to enable the Diag mode or Qualcomm DIAG port.
- Now, your device should be detected as Qualcomm HS-USB Android DIAG 901D in Device Manager.
Enable Qualcomm Diagnostic Mode using Terminal APK
- Make sure your device is rooted and have proper root permissions.
- Install Terminal Emulator app from here.
- Connect your device to PC.
- Open Terminal Emulator App and enter the below commands:
- Now, your device should be detected as Qualcomm HS-USB Android DIAG 901D in Device Manager.
Drivers Usb Config Port Devices Adapter
These are the three methods using which we can enable Diag Mode and fix cannot find the DIAG COM Port problem on Android.
Have any queries? Ask them in the comment section below.
Drivers Usb Config Port Devices Gigabit
Related
Drivers Usb Config Port Devices Usb
This page may contain affiliate links so we earn a commission. Please read our affiliate disclosure for more info.
